예제)
JDBC 프로그램을 작성하기 위해서는 JDBC 관련 라이브러리 파일(ojdbc 라이브러리)을 프로젝트에 빌드 처리해주어야 한다.
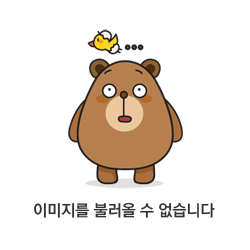
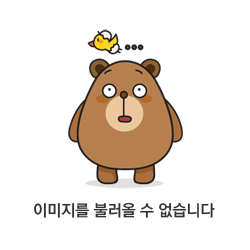
1. STUDENT 테이블의 학생정보를 저장하여 전달하기 위한 클래스 StudentDTO 클래스 선언
package xyz.itwill.dto;
/*
이름 널? 유형
-------- -------- -------------
NO NOT NULL NUMBER(4)
NAME VARCHAR2(50)
PHONE VARCHAR2(20)
ADDRESS VARCHAR2(100)
BIRTHDAY DATE
*/
//STUDENT 테이블의 학생정보를 저장하여 전달하기 위한 클래스
public class StudentDTO {
private int no;
private String name;
private String phone;
private String address;
private String birthday;
public StudentDTO() {
// TODO Auto-generated constructor stub
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
}
2. JNDI를 통해 커넥션 객체가 미리 저장된 DBCP 객체를 생성한다.
* DBCP(DataBase Connection Pool) 객체는 다수의 Connection 객체를 미리 생성하여 저장하고 제공하기 위한 객체이다. 서버에 미리 접속되어있기 때문에 속도가 매우 빠르다는 장점이 있다.
* JNDI는 WAS 프로그램에 의해 관리되는 객체를 미리 생성하여 저장하고 웹프로그램에서 필요한 경우 WAS 프로그램에 등록된 객체의 이름을 이용하여 객체를 제공받아 사용하기 위한 기능이다.
META-INF 디렉토리에 [context.xml] 파일을 생성한다. ([context.xml] 파일에는 DBCP의 설정 정보를 작성)
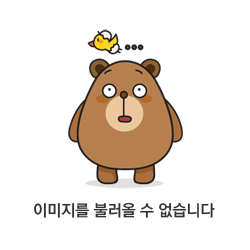
[context.xml]
<?xml version="1.0" encoding="UTF-8"?>
<Context>
<Resource name="jdbc/oracle" auth="Container"
factory="org.apache.tomcat.dbcp.dbcp2.BasicDataSourceFactory"
type="javax.sql.DataSource" driverClassName="oracle.jdbc.driver.OracleDriver"
url="jdbc:oracle:thin:@localhost:1521:xe" username="scott" password="tiger"
initialSize="10" maxIdle="10" maxTotal="15"/>
</Context>
3. JDBC 기능을 제공하는 DAO 클래스가 상속받아 사용하기 위해 작성된 부모 클래스인 JdbcDAO 클래스 선언
[JdbcDAO.java]
package xyz.itwill.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public abstract class JdbcDAO {//상속만을 목적으로 작성된 클래스
private static DataSource dataSource;
//DataSource 객체를 제공받아 필드에 저장
static {
try {
dataSource=(DataSource)new InitialContext().lookup("java:comp/env/jdbc/oracle");
} catch (NamingException e) {
e.printStackTrace();
}
}
//DataSource 객체에서 Connection 객체를 가져오는 메소드
public Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
public void close(Connection con) {
try {
if(con!=null) con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void close(Connection con, PreparedStatement pstmt) {
try {
if(pstmt!=null) pstmt.close();
if(con!=null) con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void close(Connection con, PreparedStatement pstmt, ResultSet rs) {
try {
if(rs!=null) rs.close();
if(pstmt!=null) pstmt.close();
if(con!=null) con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
4. STUDENT 테이블에 행(학생정보)에 대한 삽입, 변경, 삭제, 검색 기능을 제공하는 StudentDAO 클래스 선언
[StudentDAO.java]
package xyz.itwill.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import oracle.jdbc.proxy.annotation.Pre;
import xyz.itwill.dto.StudentDTO;
public class StudentDAO extends JdbcDAO {
private static StudentDAO _dao;
private StudentDAO() {
}
static {
_dao=new StudentDAO();
}
public static StudentDAO getDAO() {
return _dao;
}
//학생정보를 전달받아 STUDENT 테이블에 삽입하고 삽입행의 갯수를 반환하는 메소드
public int insertStudent(StudentDTO student) {
Connection con=null;
PreparedStatement pstmt=null;
int rows=0;
try {
con=getConnection();
String sql="insert into student values(?,?,?,?,?)";
pstmt=con.prepareStatement(sql);
pstmt.setInt(1, student.getNo());
pstmt.setString(2, student.getName());
pstmt.setString(3, student.getPhone());
pstmt.setString(4, student.getAddress());
pstmt.setString(5, student.getBirthday());
} catch (SQLException e) {
System.out.println("[에러]insertStudent() 메소드의 SQL 오류 = "+e.getMessage());
} finally {
close(con, pstmt);
}
return rows;
}
//학생정보를 전달받아 STUDENT 테이블에 저장된 학생정보를 변경하고 변경행의 갯수를 반환하는 메소드
public int updateStudent(StudentDTO student) {
Connection con=null;
PreparedStatement pstmt=null;
int rows=0;
try {
con=getConnection();
String sql="update student set name=?,phone=?,address=?,birthday=? where no=?";
pstmt=con.prepareStatement(sql);
pstmt.setString(1, student.getName());
pstmt.setString(2, student.getPhone());
pstmt.setString(3, student.getAddress());
pstmt.setString(4, student.getBirthday());
pstmt.setInt(5, student.getNo());
rows=pstmt.executeUpdate();
} catch (SQLException e) {
System.out.println("[에러]updateStudent() 메소드의 SQL 오류 = "+e.getMessage());
} finally {
close(con, pstmt);
}
return rows;
}
//학번을 전달받아 STUDENT 테이블에 저장된 학생정보를 삭제하고 삭제행의 갯수를 반환하는 메소드
public int deleteStudent(int no) {
Connection con=null;
PreparedStatement pstmt=null;
int rows=0;
try {
con=getConnection();
String sql="delete from student where no=?";
pstmt=con.prepareStatement(sql);
pstmt.setInt(1, no);
rows=pstmt.executeUpdate();
} catch (SQLException e) {
System.out.println("[에러]deleteStudent() 메소드의 SQL 오류 = "+e.getMessage());
} finally {
close(con, pstmt);
}
return rows;
}
//학생번호를 전달받아 STUDENT 테이블에 저장된 학생정보를 검색하여 DTO 객체로 반환하는 메소드
public StudentDTO selectStudent(int no) {
Connection con=null;
PreparedStatement pstmt=null;
ResultSet rs=null;
StudentDTO student=null;
try {
con=getConnection();
String sql="select * from student where no=?";
pstmt=con.prepareStatement(sql);
pstmt.setInt(1, no);
rs=pstmt.executeQuery();
if(rs.next()) {
student=new StudentDTO();
student.setNo(rs.getInt("no"));
student.setName(rs.getString("name"));
student.setPhone(rs.getString("phone"));
student.setAddress(rs.getString("address"));
student.setBirthday(rs.getString("birthday"));
}
} catch (SQLException e) {
System.out.println("[에러]selectStudent() 메소드의 SQL 오류 = "+e.getMessage());
} finally {
close(con, pstmt, rs);
}
return student;
}
//STUDENT 테이블에 저장된 모든 학생정보를 검색하여 List 객체로 반환하는 메소드
public List<StudentDTO> selectStudentList() {
Connection con=null;
PreparedStatement pstmt=null;
ResultSet rs=null;
List<StudentDTO> studentList=new ArrayList<>();
try {
con=getConnection();
String sql="select * from student order by no";
pstmt=con.prepareStatement(sql);
rs=pstmt.executeQuery();
while(rs.next()) {
StudentDTO student=new StudentDTO();
student.setNo(rs.getInt("no"));
student.setName(rs.getString("name"));
student.setPhone(rs.getString("phone"));
student.setAddress(rs.getString("address"));
student.setBirthday(rs.getString("birthday"));
studentList.add(student);
}
} catch (SQLException e) {
System.out.println("[에러]selectStudentList() 메소드의 SQL 오류 = "+e.getMessage());
} finally {
close(con, pstmt, rs);
}
return studentList;
}
}
5. JSP를 만들어 웹 프로그램 만들기
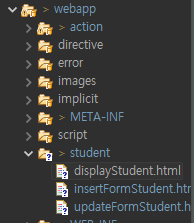
[displayStudent.html]
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JSP</title>
</head>
<body>
<h1 align="center">학생리스트</h1>
<table align="center" cellspacing="0" cellpadding="1" width="800">
<tr align="right">
<td>
<input type="button" value="학생추가">
</td>
</tr>
</table>
<table align="center" border="1" cellspacing="0" cellpadding="1" width="800">
<tr bgcolor="yellow">
<th width="100">학생번호</th>
<th width="100">이름</th>
<th width="150">전화번호</th>
<th width="250">주소</th>
<th width="100">생년월일</th>
<th width="50">삭제</th>
<th width="50">변경</th>
</tr>
<!-- 학생정보 출력-->
<tr align="center">
<td width="100">1000</td>
<td width="100">홍길동</td>
<td width="150">010-1234-5678</td>
<td width="250">서울시 강남구</td>
<td width="100">1999-12-31</td>
<td width="50"><input type="button" value="삭제"></td>
<td width="50"><input type="button" value="변경"></td>
</tr>
</table>
</body>
</html>
[insertFormStudent.html]
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JSP</title>
</head>
<body>
<h1 align="center">학생정보 입력</h1>
<hr>
<form name="studentForm">
<table align="center" border="1" cellpadding="1" cellspacing="0" width="300">
<tr height="40">
<th bgcolor="yellow" width="100">학생번호</th>
<td width="200" align="center">
<input type="text" name="no">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">이름</th>
<td width="200" align="center">
<input type="text" name="name">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">전화번호</th>
<td width="200" align="center">
<input type="text" name="phone">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">주소</th>
<td width="200" align="center">
<input type="text" name="address">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">생년월일</th>
<td width="200" align="center">
<input type="text" name="birthday">
</td>
</tr>
<tr height="40">
<td width="200" colspan="2" align="center">
<input type="button" value="학생추가" onclick="submitCheck();">
<input type="reset" value="초기화">
<input type="button" value="학생목록">
</td>
</tr>
</table>
</form>
<script type="text/javascript">
studentForm.no.focus();
function submitCheck() {
if(studentForm.no.value=="") {
alert("학생번호를 입력해 주세요.");
studentForm.no.focus();
return;
}
var noReg=/\d{4}/g;
if(!noReg.test(studentForm.no.value)) {
alert("학생번호는 정수 4자리로 입력해주세요.");
studentForm.no.focus();
return;
}
if(studentForm.name.value=="") {
alert("이름을 입력해 주세요.");
studentForm.name.focus();
return;
}
if(studentForm.phone.value=="") {
alert("전화번호을 입력해 주세요.");
studentForm.phone.focus();
return;
}
var phoneReg=/(01[016789])-\d{3,4}-\d{4}/g;
if(!phoneReg.test(studentForm.phone.value)) {
alert("전화번호를 형식에 맞게 입력해주세요.");
studentForm.phone.focus();
return;
}
if(studentForm.address.value=="") {
alert("주소을 입력해 주세요.");
studentForm.address.focus();
return;
}
if(studentForm.birthday.value=="") {
alert("생년월일을 입력해 주세요.");
studentForm.birthday.focus();
return;
}
var birthdayReg=/(18|19|20)\d{2}-(0[1-9]|1[0-2])-(0[1-9]|[12][0-9]|3[01])/g;
if(!birthdayReg.test(studentForm.birthday.value)) {
alert("생년월일을 형식에 맞게 입력해주세요.");
studentForm.birthday.focus();
return;
}
studentForm.method="POST";
studentForm.action="insertStudent.jsp";
studentForm.submit();
}
</script>
</body>
</html>
[updateFormStudent.html]
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JSP</title>
</head>
<body>
<h1 align="center">학생정보 변경</h1>
<hr>
<form name="studentForm">
<table align="center" border="1" cellpadding="1" cellspacing="0" width="300">
<tr height="40">
<th bgcolor="yellow" width="100">학생번호</th>
<td width="200" align="center">
1000
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">이름</th>
<td width="200" align="center">
<input type="text" name="name" value="홍길동">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">전화번호</th>
<td width="200" align="center">
<input type="text" name="phone" value="010-1234-5678">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">주소</th>
<td width="200" align="center">
<input type="text" name="address" value="서울시 강남구">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">생년월일</th>
<td width="200" align="center">
<input type="text" name="birthday" value="1999-12-27">
</td>
</tr>
<tr height="40">
<td width="200" colspan="2" align="center">
<input type="button" value="학생변경" onclick="submitCheck();">
<input type="reset" value="초기화">
<input type="button" value="학생목록">
</td>
</tr>
</table>
</form>
<script type="text/javascript">
studentForm.num.focus();
function submitCheck() {
if(studentForm.name.value=="") {
alert("이름을 입력해 주세요.");
studentForm.name.focus();
return;
}
if(studentForm.phone.value=="") {
alert("전화번호을 입력해 주세요.");
studentForm.phone.focus();
return;
}
if(studentForm.address.value=="") {
alert("주소을 입력해 주세요.");
studentForm.address.focus();
return;
}
if(studentForm.birthday.value=="") {
alert("생년월일을 입력해 주세요.");
studentForm.birthday.focus();
return;
}
studentForm.method="POST";
studentForm.action="updateStudent.jsp";
studentForm.submit();
}
</script>
</body>
</html>
4-1) html 문서를 jsp로 바꿔주기 (자바코드를 사용할 수 있도록)
1. 확장자 바꾸기 (.jsp)
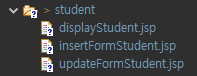
2. 상단에 page 디렉티브 삽입
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
[displayStudent.jsp]
STUDENT 테이블에 저장된 모든 학생정보를 검색하여 클라이언트에게 전달하여 응답하는 JSP 문서
→ [학생추가] 태그를 클릭한 경우 [insertFormStudent.jsp] 문서를 요청하여 이동
→ 학생정보 출력태그에서 [변경] 태그를 클릭한 경우 [updateFormStudent.jsp] 문서를 요청하여 이동 - 학생번호 전달
→ 학생정보 출력태그에서 [삭제] 태그를 클릭한 경우 [deleteStudent.jsp] 문서를 요청하여 이동 - 학생번호 전달
<%@page import="xyz.itwill.dao.StudentDAO"%>
<%@page import="xyz.itwill.dto.StudentDTO"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//STUDENT 테이블에 저장된 모든 학생정보를 검색하여 List 객체로 반환하는 DAO 클래스의 메소드 호출
List<StudentDTO> studentList=StudentDAO.getDAO().selectStudentList();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JSP</title>
</head>
<body>
<h1 align="center">학생리스트</h1>
<table align="center" cellspacing="0" cellpadding="1" width="800">
<tr align="right">
<td>
<input type="button" value="학생추가" onclick="location.href='insertFormStudent.jsp';">
</td>
</tr>
</table>
<table align="center" border="1" cellspacing="0" cellpadding="1" width="800">
<tr bgcolor="yellow">
<th width="100">학생번호</th>
<th width="100">이름</th>
<th width="150">전화번호</th>
<th width="250">주소</th>
<th width="100">생년월일</th>
<th width="50">삭제</th>
<th width="50">변경</th>
</tr>
<!-- 학생정보 출력-->
<% for(StudentDTO student : studentList) { %>
<tr align="center">
<td width="100"><%=student.getNo() %></td>
<td width="100"><%=student.getName() %></td>
<td width="150"><%=student.getPhone() %></td>
<td width="250"><%=student.getAddress() %></td>
<td width="100"><%=student.getBirthday().substring(0, 10) %></td>
<td width="50"><input type="button" value="삭제" onclick="removeConfirm(<%=student.getNo()%>);"></td>
<td width="50"><input type="button" value="변경" onclick="location.href='updateFormStudent.jsp?no=<%=student.getNo()%>';"></td>
</tr>
<% } %>
</table>
<script type="text/javascript">
function removeConfirm(no) {
if(confirm("학생정보를 정말로 삭제 하시겠습니까?")) {
location.href="deleteStudent.jsp?no="+no;
}
}
</script>
</body>
</html>
[insertFormStudent.jsp]
사용자로부터 학생정보를 입력받기 위한 JSP 문서
→ [학생추가] 태그를 클릭한 경우 [insertStudent.jsp] 문서를 요청하여 이동 - 입력값 전달
→ [학생목록] 태그를 클릭한 경우 [displayStudent.jsp] 문서를 요청하여 이동
<%@page import="xyz.itwill.dto.StudentDTO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String message=(String)session.getAttribute("message");
if(message==null) {
message="";
} else {
session.removeAttribute("message");
}
StudentDTO student=(StudentDTO)session.getAttribute("student");
if(student!=null) {
session.removeAttribute("student");
}
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JSP</title>
</head>
<body>
<h1 align="center">학생정보 입력</h1>
<hr>
<form name="studentForm">
<table align="center" border="1" cellpadding="1" cellspacing="0" width="300">
<tr height="40">
<th bgcolor="yellow" width="100">학생번호</th>
<td width="200" align="center">
<input type="text" name="no" <% if(student!=null) { %>value="<%=student.getNo() %>"<% } %>>
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">이름</th>
<td width="200" align="center">
<input type="text" name="name" <% if(student!=null) { %>value="<%=student.getName() %>"<% } %>>
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">전화번호</th>
<td width="200" align="center">
<input type="text" name="phone" <% if(student!=null) { %>value="<%=student.getPhone() %>"<% } %>>
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">주소</th>
<td width="200" align="center">
<input type="text" name="address" <% if(student!=null) { %>value="<%=student.getAddress() %>"<% } %>>
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">생년월일</th>
<td width="200" align="center">
<input type="text" name="birthday" <% if(student!=null) { %>value="<%=student.getBirthday() %>"<% } %>>
</td>
</tr>
<tr height="40">
<td width="200" colspan="2" align="center">
<input type="button" value="학생추가" onclick="submitCheck();">
<input type="reset" value="초기화">
<input type="button" value="학생목록" onclick="location.href='displayStudent.jsp';">
</td>
</tr>
</table>
</form>
<p align="center" style="color: red;"><%=message %></p>
<script type="text/javascript">
studentForm.no.focus();
function submitCheck() {
if(studentForm.no.value=="") {
alert("학생번호를 입력해 주세요.");
studentForm.no.focus();
return;
}
var noReg=/\d{4}/g;
if(!noReg.test(studentForm.no.value)) {
alert("학생번호는 정수 4자리로 입력해주세요.");
studentForm.no.focus();
return;
}
if(studentForm.name.value=="") {
alert("이름을 입력해 주세요.");
studentForm.name.focus();
return;
}
if(studentForm.phone.value=="") {
alert("전화번호를 입력해 주세요.");
studentForm.phone.focus();
return;
}
var phoneReg=/(01[016789])-\d{3,4}-\d{4}/g;
if(!phoneReg.test(studentForm.phone.value)) {
alert("전화번호를 형식에 맞게 입력해주세요.");
studentForm.phone.focus();
return;
}
if(studentForm.address.value=="") {
alert("주소를 입력해 주세요.");
studentForm.address.focus();
return;
}
if(studentForm.birthday.value=="") {
alert("생년월일을 입력해 주세요.");
studentForm.birthday.focus();
return;
}
var birthdayReg=/(18|19|20)\d{2}-(0[1-9]|1[0-2])-(0[1-9]|[12][0-9]|3[01])/g;
if(!birthdayReg.test(studentForm.birthday.value)) {
alert("생년월일을 형식에 맞게 입력해주세요.");
studentForm.birthday.focus();
return;
}
studentForm.method="post";
studentForm.action="insertStudent.jsp";
studentForm.submit();
}
</script>
</body>
</html>
[insertStudent.jsp]
학생정보를 전달받아 STUDENT 테이블의 행으로 삽입하고 [displayStudent.jsp] 문서를 요청할 수 있는 URL 주소를 클라이언트에게 전달하여 응답하는 JSP 문서
<%@page import="xyz.itwill.dto.StudentDTO"%>
<%@page import="xyz.itwill.dao.StudentDAO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%-- 학생정보를 전달받아 STUDENT 테이블의 행으로 삽입하고 [displayStudent.jsp] 문서를 요청할
수 있는 URL 주소를 클라이언트에게 전달하여 응답하는 JSP 문서 --%>
<%
//JSP 문서를 GET 방식으로 요청한 경우 - 비정상적인 요청
if(request.getMethod().equals("GET")) {
session.setAttribute("message", "비정상적인 방법으로 페이지를 요청 하였습니다.");
response.sendRedirect("insertFormStudent.jsp");//클라이언트에게 URL 주소 전달
return;
}
//POST 방식으로 요청하여 전달된 값에 대한 캐릭터셋 변경
request.setCharacterEncoding("utf-8");
//전달값을 반환받아 변수에 저장
int no=Integer.parseInt(request.getParameter("no"));
String name=request.getParameter("name");
String phone=request.getParameter("phone");
String address=request.getParameter("address");
String birthday=request.getParameter("birthday");
//DTO 클래스로 객체를 생성하여 전달값으로 필드값 변경
StudentDTO student=new StudentDTO();
student.setNo(no);
student.setName(name);
student.setPhone(phone);
student.setAddress(address);
student.setBirthday(birthday);
//사용자로부터 입력받아 전달된 학생번호가 STUDENT 테이블에 저장된 기존 학생정보의 학생번호와
//중복될 경우 [insertFormStudent.jsp] 문서로 이동할 수 있는 URL 주소를 클라이언트에게 전달
//학생번호를 전달받아 STUDENT 테이블에 저장된 학생정보를 검색하여 DTO 객체로 반환하는 DAO 클래스의 메소드 호출
// => null 반환 : 학생정보 미검색 - 학생번호 미중복, StudentDTO 객체 반환 : 학생정보 검색 - 학생번호 중복
if(StudentDAO.getDAO().selectStudent(no)!=null) {//학생번호가 중복된 경우
session.setAttribute("message", "이미 사용중인 학생번호를 입력 하였습니다. 다시 입력해 주세요.");
session.setAttribute("student", student);
response.sendRedirect("insertFormStudent.jsp");//클라이언트에게 URL 주소 전달
return;
}
//학생정보를 전달받아 STUDENT 테이블의 행으로 삽입하는 DAO 클래스의 메소드 호출
StudentDAO.getDAO().insertStudent(student);
//클라이언트에게 URL 주소 전달
response.sendRedirect("displayStudent.jsp");
%>
[updateFormStudent.jsp]
학생번호를 전달받아 STUDENT 테이블에 저장된 학생정보를 검색하여 입력태그의 초기값으로 출력하고 변경값을 입력받기 위한 JSP 문서
→ [학생변경] 태그를 클릭한 경우 [updateStudent.jsp] 문서를 요청하여 이동 - 입력값(학생정보) 전달
→ [학생목록] 태그를 클릭한 경우 [displayStudent.jsp] 문서를 요청하여 이동
<%@page import="xyz.itwill.dao.StudentDAO"%>
<%@page import="xyz.itwill.dto.StudentDTO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//전달값이 없는 경우 - 비정상적인 요청
if(request.getParameter("no")==null) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST);
return;
}
//전달값을 반환받아 변수에 저장
int no=Integer.parseInt(request.getParameter("no"));
//학생번호를 전달받아 STUDENT 테이블에 저장된 학생정보를 검색하여 DTO 객체로 반환하는 DAO 클래스의 메소드 호출
StudentDTO student=StudentDAO.getDAO().selectStudent(no);
//STUDENT 테이블에 저장된 학생정보를 검색하지 못한 경우 - 비정상적인 요청
if(student==null) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST);
return;
}
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JSP</title>
</head>
<body>
<h1 align="center">학생정보 변경</h1>
<hr>
<%-- [updateStudent.jsp] 문서를 요청할 때 학생번호도 전달되도록 설정 --%>
<%-- => 학생번호는 변경하지 못하도록 hidden Type으로 전달하거나 입력태그의 초기값을
변경하지 못하도록 readonly 속성 사용 --%>
<form name="studentForm">
<%-- <input type="hidden" name="no" value="<%=student.getNo() %>"> --%>
<table align="center" border="1" cellpadding="1" cellspacing="0" width="300">
<tr height="40">
<th bgcolor="yellow" width="100">학생번호</th>
<td width="200" align="center">
<%-- <%=student.getNo()%> --%>
<input type="text" name="no" value="<%=student.getNo() %>" readonly="readonly">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">이름</th>
<td width="200" align="center">
<input type="text" name="name" value="<%=student.getName() %>">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">전화번호</th>
<td width="200" align="center">
<input type="text" name="phone" value="<%=student.getPhone() %>">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">주소</th>
<td width="200" align="center">
<input type="text" name="address" value="<%=student.getAddress() %>">
</td>
</tr>
<tr height="40">
<th bgcolor="yellow" width="100">생년월일</th>
<td width="200" align="center">
<input type="text" name="birthday" value="<%=student.getBirthday().substring(0, 10) %>">
</td>
</tr>
<tr height="40">
<td width="200" colspan="2" align="center">
<input type="button" value="학생변경" onclick="submitCheck();">
<input type="reset" value="초기화">
<input type="button" value="학생목록" onclick="location.href='displayStudent.jsp';">
</td>
</tr>
</table>
</form>
<script type="text/javascript">
function submitCheck() {
if(studentForm.name.value=="") {
alert("이름을 입력해 주세요.");
studentForm.name.focus();
return;
}
if(studentForm.phone.value=="") {
alert("전화번호를 입력해 주세요.");
studentForm.phone.focus();
return;
}
var phoneReg=/(01[016789])-\d{3,4}-\d{4}/g;
if(!phoneReg.test(studentForm.phone.value)) {
alert("전화번호를 형식에 맞게 입력해주세요.");
studentForm.phone.focus();
return;
}
if(studentForm.address.value=="") {
alert("주소를 입력해 주세요.");
studentForm.address.focus();
return;
}
if(studentForm.birthday.value=="") {
alert("생년월일을 입력해 주세요.");
studentForm.birthday.focus();
return;
}
var birthdayReg=/(18|19|20)\d{2}-(0[1-9]|1[0-2])-(0[1-9]|[12][0-9]|3[01])/g;
if(!birthdayReg.test(studentForm.birthday.value)) {
alert("생년월일을 형식에 맞게 입력해주세요.");
studentForm.birthday.focus();
return;
}
studentForm.method="post";
studentForm.action="updateStudent.jsp";
studentForm.submit();
}
</script>
</body>
</html>
[updateStudent.jsp]
학생정보를 전달받아 STUDENT 테이블에 저장된 학생정보를 변경하고 [displayStudent.jsp] 문서를 요청할 수 있는 URL 주소를 클라이언트에게 전달하여 응답하는 JSP 문서
<%@page import="xyz.itwill.dao.StudentDAO"%>
<%@page import="xyz.itwill.dto.StudentDTO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//JSP 문서를 GET 방식으로 요청한 경우 - 비정상적인 요청
if(request.getMethod().equals("GET")) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST);
return;
}
//POST 방식으로 요청하여 전달된 값에 대한 캐릭터셋 변경
request.setCharacterEncoding("utf-8");
//전달값을 반환받아 변수에 저장
int no=Integer.parseInt(request.getParameter("no"));
String name=request.getParameter("name");
String phone=request.getParameter("phone");
String address=request.getParameter("address");
String birthday=request.getParameter("birthday");
//DTO 클래스로 객체를 생성하여 전달값으로 필드값 변경
StudentDTO student=new StudentDTO();
student.setNo(no);
student.setName(name);
student.setPhone(phone);
student.setAddress(address);
student.setBirthday(birthday);
//학생정보를 전달받아 STUDENT 테이블에 저장된 학생정보를 변경하는 DAO 클래스의 메소드 호출
StudentDAO.getDAO().updateStudent(student);
//클라이언트에게 URL 주소 전달
response.sendRedirect("displayStudent.jsp");
%>
[deleteStudent.jsp]
학생번호를 전달받아 STUDENT 테이블에 저장된 학생정보를 삭제하고 [displayStudent.jsp] 문서를 요청할 수 있는 URL 주소를 클라이언트에게 전달하여 응답하는 JSP 문서
<%@page import="xyz.itwill.dao.StudentDAO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//전달값이 없는 경우 - 비정상적인 요청
if(request.getParameter("no")==null) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST);
return;
}
//전달값을 반환받아 변수에 저장
int no=Integer.parseInt(request.getParameter("no"));
//학생번호를 전달받아 STUDENT 테이블에 저장된 학생정보를 삭제하는 DAO 클래스의 메소드 호출
int rows=StudentDAO.getDAO().deleteStudent(no);
if(rows > 0) {//삭제행이 있는 경우 - 정상적인 요청
response.sendRedirect("displayStudent.jsp");
} else {//삭제행이 없는 경우 - 비정상적인 요청
response.sendError(HttpServletResponse.SC_BAD_REQUEST);
}
%>
'학원 > 복기' 카테고리의 다른 글
[AJAX] GET/POST 방식 요청 (0) | 2023.07.04 |
---|---|
[AJAX] AJAX란? / 예제 (0) | 2023.07.03 |
[JSP] 자바빈(JavaBean) / useBean 태그 (0) | 2023.06.26 |
[JSP] 표준 액션 태그(Standard Action Tag) - include 태그/forward 태그/param 태그 (0) | 2023.06.25 |
[JSP] 객체의 사용범위(Scope) (0) | 2023.06.25 |